📝내가 만든 좋아요 API 기능 구현하기
로그인한 유저가 게시글의 좋아요 버튼을 누르면 좋아요 버튼에 색이 입력게 구현했다
리사이클러뷰의 화면 구성은 아래 이미지처럼 만들었다
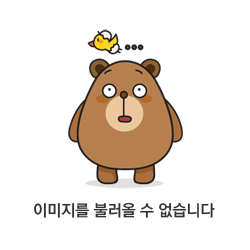
더보기
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<androidx.cardview.widget.CardView
android:id="@+id/cardView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="15dp"
android:layout_marginTop="7dp"
android:layout_marginRight="15dp"
android:layout_marginBottom="7dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="10dp"
android:orientation="horizontal">
<ImageView
android:id="@+id/imgPhoto"
android:layout_width="150dp"
android:layout_height="150dp"
android:scaleType="centerCrop"
app:srcCompat="@drawable/outline_photo_24" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginTop="20dp"
android:orientation="vertical">
<TextView
android:id="@+id/txtContent"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="TextView"
android:textColor="#000000"
android:textSize="18sp" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="TextView"
android:textColor="#000000"
android:textSize="14sp" />
<TextView
android:id="@+id/txtCreatedAt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="TextView"
android:textColor="#000000"
android:textSize="14sp" />
<ImageView
android:id="@+id/imgLike"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
app:srcCompat="@drawable/ic_thumb_up_1" />
</LinearLayout>
</LinearLayout>
</androidx.cardview.widget.CardView>
</LinearLayout>
리사이클러뷰에 표시하는것이기 때문에 어댑터쪽에 코드를 입력해도 되지만 메인 액티비티에 함수를 만들어 호출해 사용했다
api 인터페이스 코드
// 포스팅 좋아요
@POST("/like/{postingId}")
Call<Res> setLike(@Path("postingId") int postingId, @Header("Authorization") String token);
// 포스팅 좋아요 삭제
@DELETE("/like/{postingId}")
Call<Res> deleteLike(@Path("postingId") int postingId, @Header("Authorization") String token);
어댑터에 이미지를 클릭하는 이벤트 작성
imgLike.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// 1. 어느번째의 데이터의 좋아요를 누른 것인지 확인해서 함수 호출
int index = getAdapterPosition();
((MainActivity)context).likeProcess(index);
}
});
메인 액티비티에 public으로 함수 생성
public void likeProcess(int index) {
selectedPosting = postingList.get(index);
// 2. 해당 행의 좋아요가 이미 좋아요인지 아닌지 파악
if (selectedPosting.getIsLike() == 0) {
Retrofit retrofit = NetworkClient.getRetrofitClient(MainActivity.this);
PostingApi api = retrofit.create(PostingApi.class);
SharedPreferences sp = getSharedPreferences(Config.PREFERENCE_NAME, MODE_PRIVATE);
String accessToken = "Bearer " + sp.getString(Config.ACCESS_TOKEN, "");
Call<Res> call = api.setLike(selectedPosting.getPostingId(), accessToken);
call.enqueue(new Callback<Res>() {
@Override
public void onResponse(Call<Res> call, Response<Res> response) {
if (response.isSuccessful()) {
// 4. 화면에 결과를 표시
selectedPosting.setIsLike(1);
adapter.notifyDataSetChanged();
} else {
}
}
@Override
public void onFailure(Call<Res> call, Throwable t) {
}
});
} else {
// 좋아요 해제 API 호출
Retrofit retrofit = NetworkClient.getRetrofitClient(MainActivity.this);
PostingApi api = retrofit.create(PostingApi.class);
SharedPreferences sp = getSharedPreferences(Config.PREFERENCE_NAME, MODE_PRIVATE);
String accessToken = "Bearer " + sp.getString(Config.ACCESS_TOKEN, "");
Call<Res> call = api.deleteLike(selectedPosting.getPostingId(), accessToken);
call.enqueue(new Callback<Res>() {
@Override
public void onResponse(Call<Res> call, Response<Res> response) {
if (response.isSuccessful()) {
// 4. 화면에 결과를 표시
selectedPosting.setIsLike(0);
adapter.notifyDataSetChanged();
} else {
}
}
@Override
public void onFailure(Call<Res> call, Throwable t) {
}
});
}
}
'Android Studio' 카테고리의 다른 글
[Android Studio] TabBar Fragment 사용하기 (0) | 2023.02.15 |
---|---|
[Android Studio] TabBar 만들기 (0) | 2023.02.15 |
[Android Studio] UTC 시간 Local Time으로 변경하기 (0) | 2023.02.14 |
[Android Studio] Retrofit으로 API호출할때 form-data 처리하기 (0) | 2023.02.14 |
[Android Studio] AlertDialog array로 사용하기 (0) | 2023.02.13 |
댓글